Playwright Cheat Sheet for Test Automation Enthusiasts
- Yogesh Sharma
- Dec 2, 2024
- 2 min read
This cheat sheet is your quick guide to mastering Playwright, the modern end-to-end testing framework for web applications. Whether you're setting up, interacting with elements, or handling advanced features, this guide has you covered!
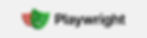
Basic Setup
Install Playwright
npm i playwright
Launch Browser
const { chromium } = require('@playwright/test');
const browser = await chromium.launch();
Create Page Instance
const context = await browser.newContext();
const page = await context.newPage();
Close Browser
await browser.close();
Basic Interactions
Navigate to a URL
await page.goto('https://example.com');
Fill a Text Field
await page.fill('input[name="example"]', 'Sample text');
Click an Element
await page.click('selector');
Select an Option
await page.selectOption('select[name="example"]', 'value');
Assertions
Expect Title
await expect(page).toHaveTitle('Expected Title');
Expect Element Visible
await expect(page.locator('selector')).toBeVisible();
Expect Text
await expect(page.locator('selector')).toHaveText('Expected Text');
Expect Attribute
await expect(page.locator('selector')).toHaveAttribute('name', 'value');
Waiting Mechanisms
Wait for Navigation
await page.waitForNavigation();
Wait for Timeout
await page.waitForTimeout(1000); // in milliseconds
Wait for Selector
await page.waitForSelector('selector');
Wait for Function
await page.waitForFunction(() => window.status === 'ready');
Working with Elements
Get Element Text
const text = await page.textContent('selector');
Get Attribute Value
const attr = await page.getAttribute('selector', 'attribute');
Take Screenshot of an Element
await page.locator('selector').screenshot({ path: 'screenshot.jpg' });
Upload Files
await page.setInputFiles('input[type="file"]', 'path/to/file');
Advanced Interactions
Execute JavaScript
const result = await page.evaluate(() => { // Custom JS code });
Emulate Devices
const { devices } = require('@playwright/test');
const iPhone = devices['iPhone 6'];
const context = await browser.newContext({ ...iPhone });
Handle Dialogs
page.on('dialog', async dialog => { await dialog.accept(); });
Intercept Network Requests
await page.route('*/', route => { // Handle the request });
This cheat sheet is ideal for anyone starting with Playwright or looking to enhance their skills. For more detailed documentation and updates, visit the official Playwright documentation.
Keep testing, keep succeeding!